Read Every Line in a Text File Python
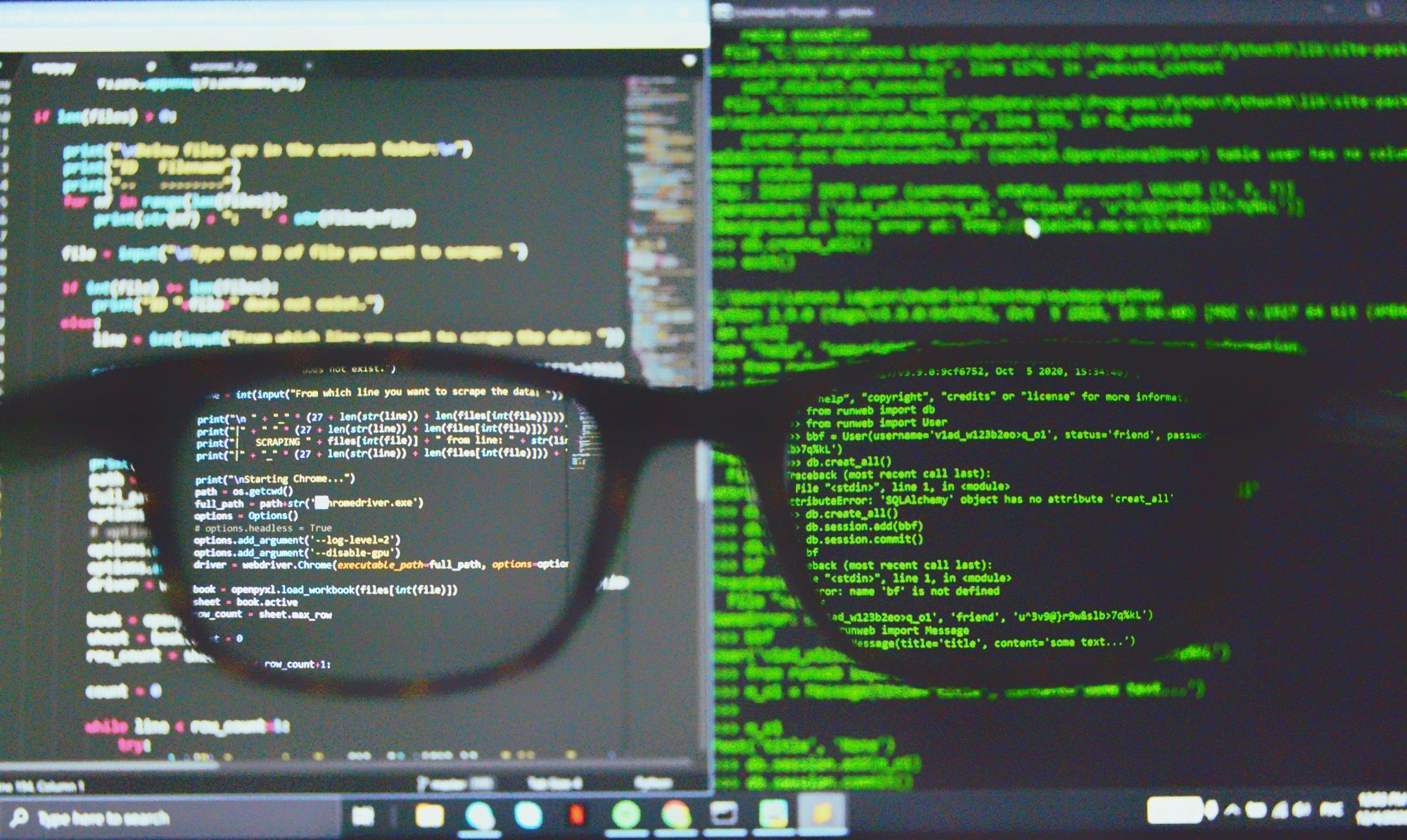
In Python, there are a few ways you can read a text file.
In this article, I will go over the open()
part, the read()
, readline()
, readlines()
, shut()
methods, and the with
keyword.
What is the open() function in Python?
If y'all want to read a text file in Python, y'all offset have to open it.
This is the bones syntax for Python's open()
function:
open("name of file you desire opened", "optional mode")
File names and correct paths
If the text file and your current file are in the same directory ("folder"), then you tin can simply reference the file name in the open()
part.
open("demo.txt")
Here is an example of both files being in the same directory:
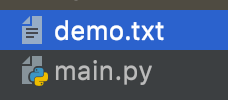
If your text file is in a different directory, then you volition need to reference the correct path name for the text file.
In this example, the random-text
file is inside a different binder then master.py
:
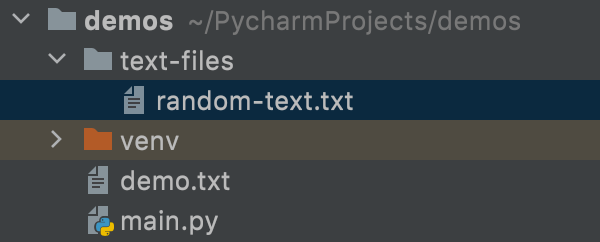
In guild to access that file in the main.py
, you accept to include the folder proper name with the name of the file.
open("text-files/random-text.txt")
If you don't have the right path for the file, and so you volition get an error message similar this:
open up("random-text.txt")

It is really important to keep track of which directory you are in and then you can reference the correct path proper name.
Optional Fashion parameter in open()
In that location are dissimilar modes when yous are working with files. The default fashion is the read mode.
The letter r
stands for read mode.
open up("demo.txt", way="r")
You can too omit mode=
and simply write "r"
.
open("demo.txt", "r")
There are other types of modes such equally "west"
for writing or "a"
for appending. I am not going to go into detail for the other modes considering we are but going to focus on reading files.
For a complete list of the other modes, delight read through the documentation.
Additional parameters for the open()
part in Python
The open up()
office can take in these optional parameters.
- buffering
- encoding
- errors
- newline
- closefd
- opener
To larn more about these optional parameters, please read through the documentation.
What is the readable() method in Python?
If you lot want to check if a file can be read, then you tin utilize the readable()
method. This will return a True
or False
.
This example would render Truthful
because nosotros are in the read mode:
file = open("demo.txt") impress(file.readable())
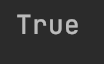
If I changed this example, to "w"
(write) mode, and then the readable()
method would render Imitation
:
file = open("demo.txt", "w") print(file.readable())
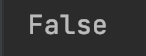
What is the read() method in Python?
The read()
method is going to read all of the content of the file equally ane string. This is a good method to use if you don't accept a lot of content in the text file.
In this example, I am using the read()
method to print out a list of names from the demo.txt
file:
file = open("demo.txt") print(file.read())

This method can accept in an optional parameter called size. Instead of reading the whole file, only a portion of it will be read.
If we modify the earlier example, we can print out only the first word by calculation the number 4 as an argument for read()
.
file = open("demo.txt") print(file.read(4))

If the size argument is omitted, or if the number is negative, then the whole file volition be read.
What is the shut() method in Python?
Once you are done reading a file, it is important that you close information technology. If you forget to close your file, then that can cause issues.
This is an example of how to close the demo.txt
file:
file = open("demo.txt") impress(file.read()) file.close()
How to use the with
keyword to close files in Python
I mode to ensure that your file is closed is to use the with
keyword. This is considered adept practise, considering the file volition shut automatically instead of you having to manually shut it.
Here is how to rewrite our example using the with
keyword:
with open("demo.txt") as file: impress(file.read())
What is the readline() method in Python?
This method is going to read one line from the file and return that.
In this case, we take a text file with these two sentences:
This is the offset line This is the second line
If we employ the readline()
method, it will just impress the outset sentence of the file.
with open("demo.txt") as file: impress(file.readline())

This method likewise takes in the optional size parameter. We tin can modify the example to add together the number vii to only read and print out This is
:
with open up("demo.txt") every bit file: print(file.readline(7))
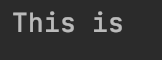
What is the readlines() method in Python?
This method volition read and return a listing of all of the lines in the file.
In this case, we are going to print out our grocery items equally a list using the readlines()
method.
with open("demo.txt") every bit file: print(file.readlines())

How to use a for loop to read lines from a file in Python
An alternative to these unlike read methods would exist to apply a for loop
.
In this case, we tin print out all of the items in the demo.txt
file by looping over the object.
with open("demo.txt") as file: for item in file: impress(particular)

Decision
If y'all want to read a text file in Python, you first have to open it.
open("name of file you want opened", "optional manner")
If the text file and your current file are in the same directory ("binder"), then you lot can just reference the file name in the open up()
function.
If your text file is in a unlike directory, and so you will need to reference the right path proper noun for the text file.
The open up()
function takes in the optional mode parameter. The default style is the read mode.
open("demo.txt", "r")
If you want to check if a file tin can be read, then you lot can use the readable()
method. This will return a True
or False
.
file.readable()
The read()
method is going to read all of the content of the file equally one string.
file.read()
Once you are done reading a file, it is important that you close it. If yous forget to shut your file, then that can cause problems.
file.shut()
One way to ensure that your file is airtight is to utilize the with
keyword.
with open("demo.txt") as file: impress(file.read())
The readline()
method is going to read 1 line from the file and return that.
file.readline()
The readlines()
method will read and return a list of all of the lines in the file.
file.readlines()
An alternative to these different read methods would be to apply a for loop
.
with open("demo.txt") as file: for detail in file: print(particular)
I hope y'all enjoyed this commodity and all-time of luck on your Python journey.
Acquire to code for free. freeCodeCamp's open source curriculum has helped more than xl,000 people become jobs every bit developers. Get started
Source: https://www.freecodecamp.org/news/python-open-file-how-to-read-a-text-file-line-by-line/
0 Response to "Read Every Line in a Text File Python"
Post a Comment